
30 Basic Conditional Statement Programming Questions in C++
Q 1. Write a program to check whether a number is positive or negative.
Input: 10
Expected Output: Positive
Q 2. Write a program to check if a number is even or odd.
Input: 7
Expected Output: Odd
Q 3. Write a program to find the maximum of two numbers.
Input: 12, 25
Expected Output: 25
Q 4. Write a program to check if a number is divisible by 5.
Input: 20
Expected Output: Divisible by 5
Q 5. Write a program to check whether a year is a leap year.
Input: 2020
Expected Output: Leap Year
Q 6. Write a program to find the largest of three numbers.
Input: 10, 30, 25
Expected Output: 30
Q 7. Write a program to check if a number is a multiple of both 3 and 5.
Input: 15
Expected Output: Multiple of Both
Q 8. Write a program to check whether a character is an alphabet.
Input: a
Expected Output: Alphabet
Q 9. Write a program to check whether a character is a vowel or consonant.
Input: e
Expected Output: Vowel
Q 10. Write a program to find the grade of a student based on marks.
Input: 85
Expected Output: A
Q 11. Write a program to check if a number is zero.
Input: 0
Expected Output: Zero
Q 12. Write a program to determine whether a person is a child, teenager, or adult based on their age.
Input: 16
Expected Output: Teenager
Q 13. Write a program to check whether a character is uppercase or lowercase.
Input: G
Expected Output: Uppercase
Q 14. Write a program to check whether a number is prime.
Input: 7
Expected Output: Prime
Q 15. Write a program to check whether a number is an Armstrong number.
Input: 153
Expected Output: Armstrong
Q 16. Write a program to calculate whether there is a profit or loss from given cost and selling price.
Input: 50, 75
Expected Output: Profit
Q 17. Write a program that accepts a number (1-7) and prints the corresponding day of the week.
Input: 3
Expected Output: Wednesday
Q 18. Write a program to check if three sides form a valid triangle.
Input: 3, 4, 5
Expected Output: Valid Triangle
Q 19. Write a program to check if a number is positive, negative, or zero.
Input: 0
Expected Output: Zero
Q 20. Write a program to check if a character is a digit.
Input: 5
Expected Output: Digit
Q 21. Write a program to check if a character is a special symbol.
Input: #
Expected Output: Special Symbol
Q 22. Write a program to check if a given year is a century year.
Input: 1900
Expected Output: Century Year
Q 23. Write a program to check if a person is eligible to vote (age >= 18).
Input: 20
Expected Output: Eligible
Q 24. Write a program to check whether given time in 24-hour format is AM or PM.
Input: 13
Expected Output: PM
Q 25. Write a program to check if a number is a palindrome.
Input: 121
Expected Output: Palindrome
Q 26. Write a program to check if a person is a senior citizen (age >= 60).
Input: 65
Expected Output: Senior Citizen
Q 27. Write a program to find the smaller of two numbers using the ternary operator.
Input: 12, 20
Expected Output: 12
Q 28. Write a program to check if two numbers are equal or not.
Input: 10, 10
Expected Output: Equal
Q 29. Write a program to check if a number is in the range of 10 to 20 (inclusive).
Input: 15
Expected Output: In Range
Q 30. Write a program to check if a given year is before or after the year 2000.
Input: 1999
Expected Output: Before 2000
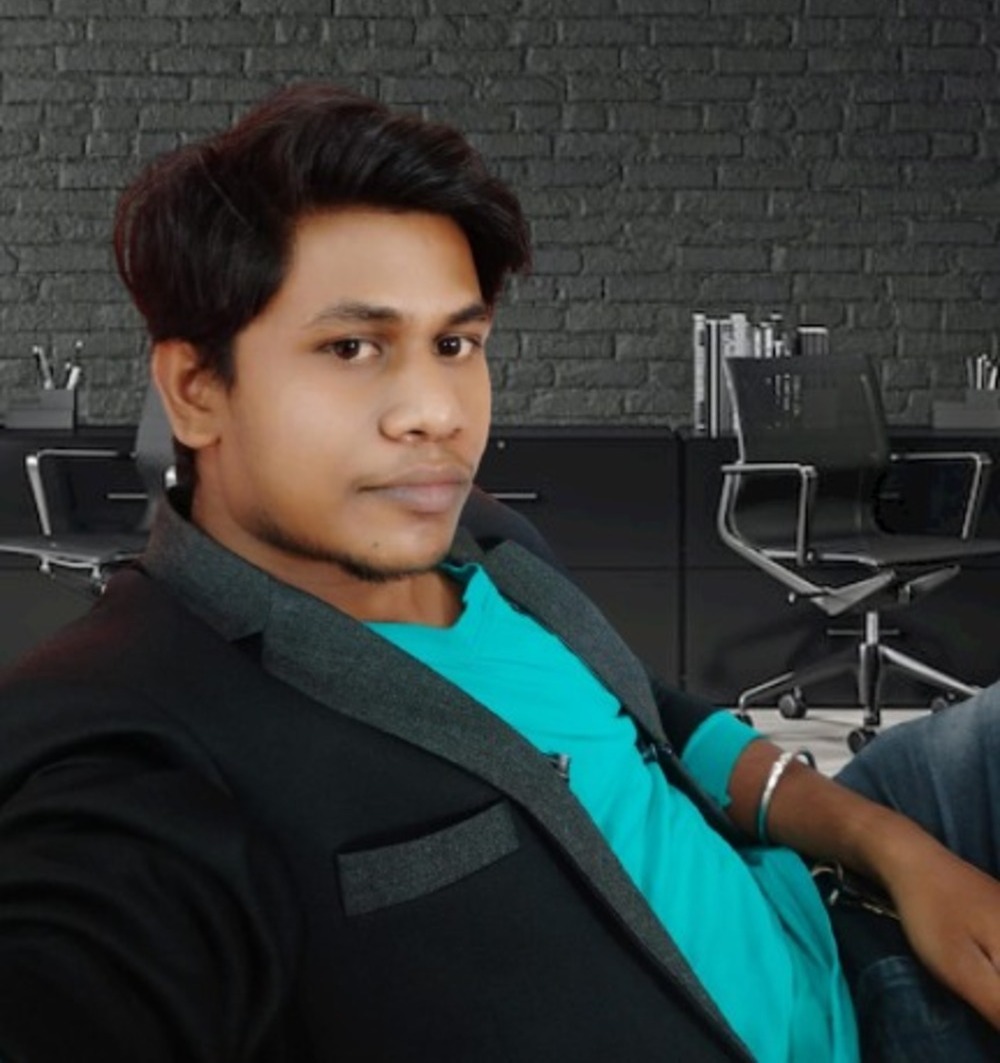
Bikki Singh
Hi, I am the instructor of TechnoVlogs. I have a strong love for programming and enjoy teaching through practical examples. I made this site to help people improve their coding skills by solving real-world problems. With years of experience, my goal is to make learning programming easy and fun for everyone. Let's learn and grow together!